Loops
The engine supports Loop Mode for the following nodes:
- Expression Node
- Decision Table Node
- Sub-Decision Node
When Loop Mode is enabled, and the input to the node is an array, the node will evaluate each element of the array separately and output an array of results.
How loop mode works
With Loop Mode:
- The node takes an array as input.
- It processes each element in the array individually.
- It outputs an array where each element corresponds to the result of evaluating one element of the input array.
Example scenario
Consider the following input to the node:
{
"customer": {
"name": "John Doe",
"country": "US"
},
"items": [
{
"name": "Laptop",
"amount": 1200,
"group": "electronics"
},
{
"name": "Mouse",
"amount": 25,
"group": "accessories"
}
]
}
We want to create a graph that applies a discount to each item if it meets specific conditions. For instance, if an item belongs to the "accessories" group and its amount is greater than 20, a discount of 5 is set.
First, we’ll set up the graph as shown below:
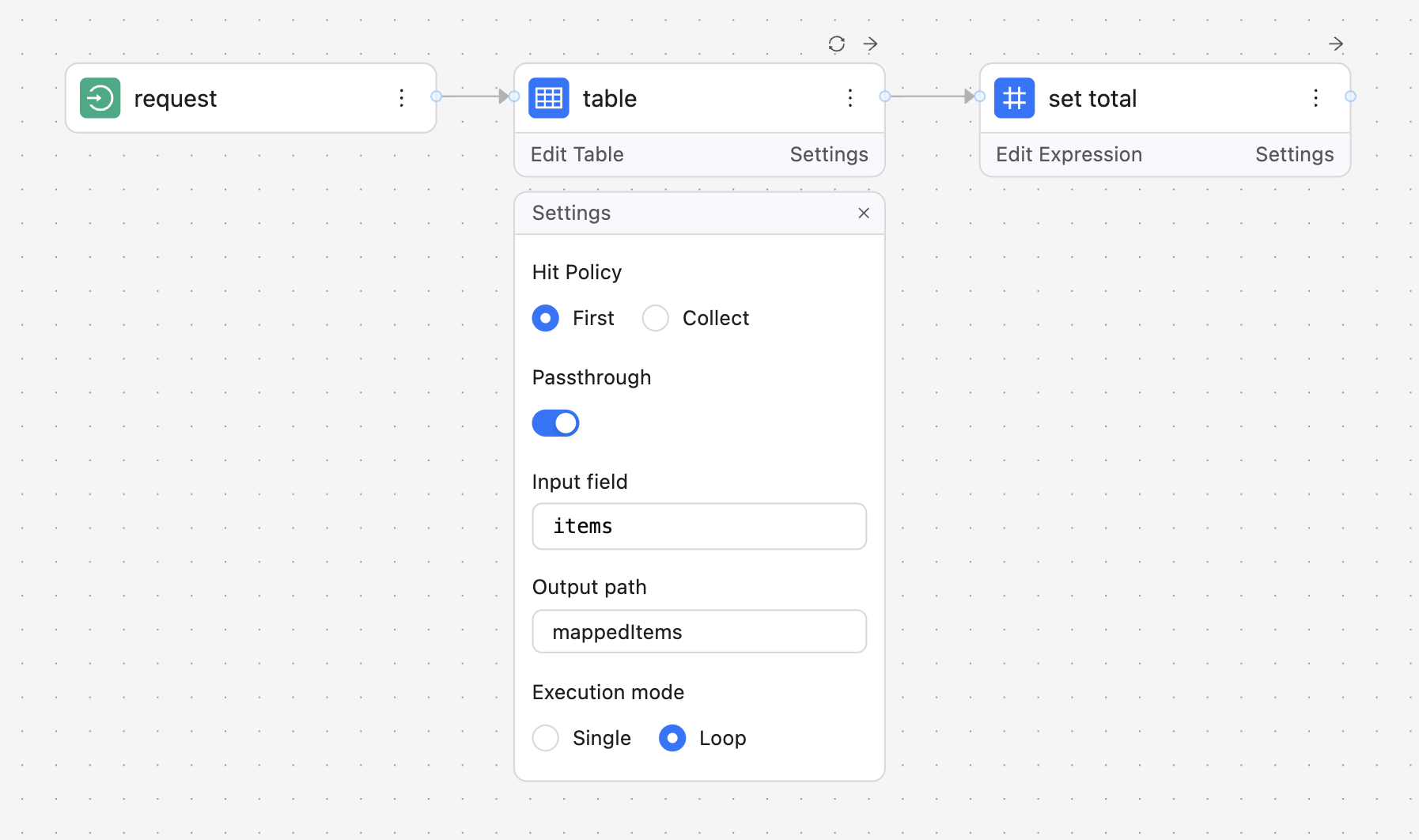
Next, we enable Loop Mode for the table, set items
as the input, and use mappedItems
as the output path.
By adding rules such as:
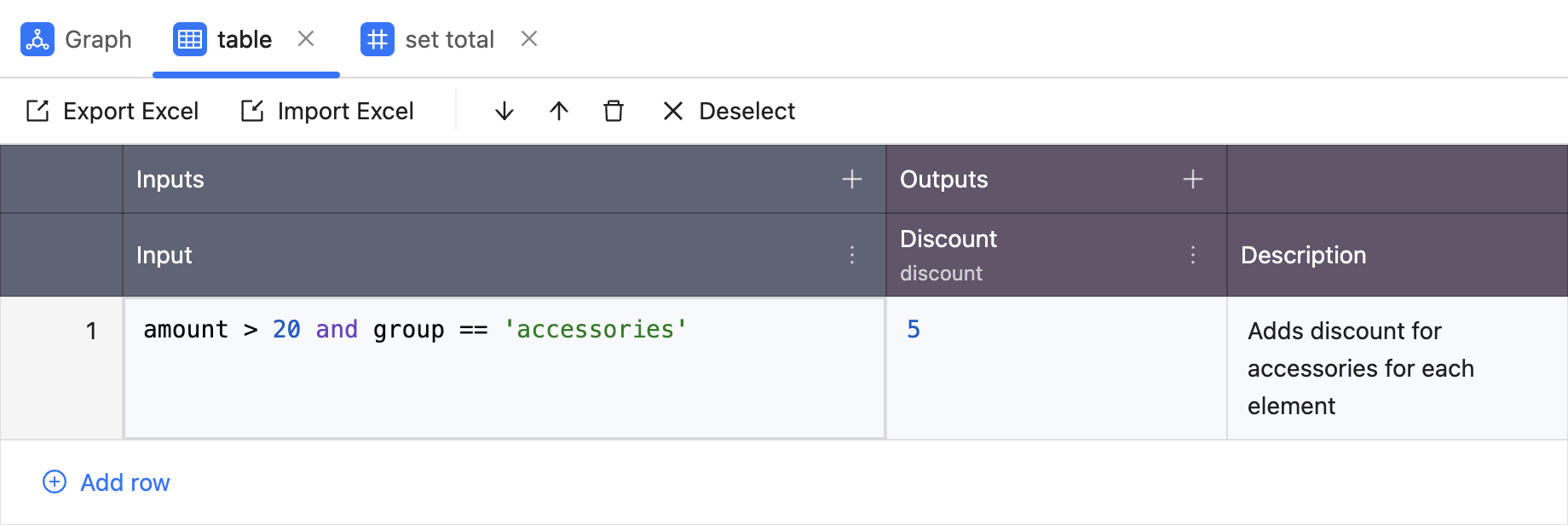
The table node will evaluate each item
individually, resulting in:
{
"customer": {}, // more data here
"items": [], // more data here
"mappedItems": [
{ "name": "Laptop", "group": "electronics", "amount": 1200 },
{ "name": "Mouse", "group": "accessories", "amount": 25, "discount": 5 }
]
}
Following this, we calculate the total amount of the mapped items, the total discount, and set the final total on the cart.
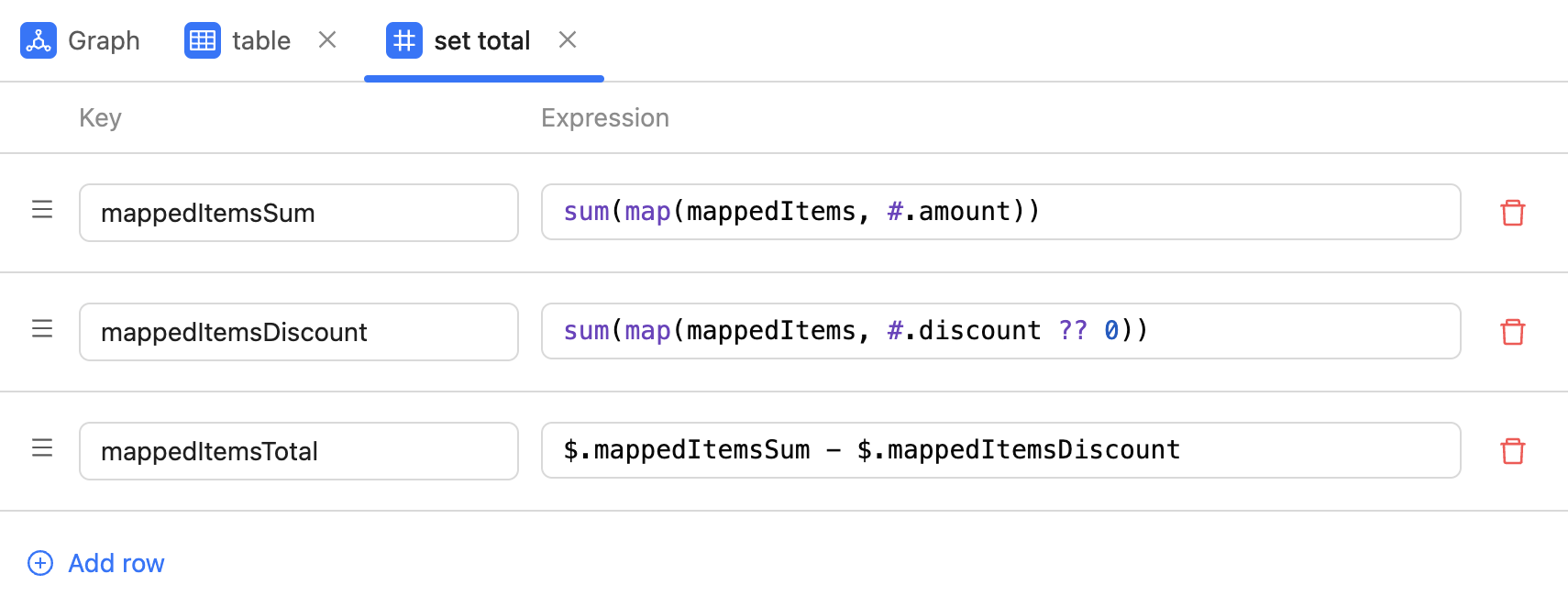
Final output will look like:
{
"customer": {}, // more data goes here
"items": [], // more data goes here
"mappedItems": [
{
"amount": 1200,
"group": "electronics",
"name": "Laptop"
},
{
"amount": 25,
"discount": 5,
"group": "accessories",
"name": "Mouse"
}
],
"mappedItemsDiscount": 5,
"mappedItemsSum": 1225,
"mappedItemsTotal": 1220
}
Global context in loops
If you need to access fields outside of a specific item, you can use the $nodes
global context. For example, to create a rule that checks if the group
is "accessory," the amount
is greater than 20, and the customer's country
is "US," you would write:
group == 'accessory' and amount > 20 and $nodes.request.customer.country == 'US'
Additional properties
Two additional properties enhance the flexibility of Loop Mode:
Input Field
The Input Field allows you to specify which field from the input should be looped over and evaluated by the node. Additionally, it supports full GoRules expressions, enabling preprocessing of data before evaluation.
Example 1: Basic Usage
Given the input:
{
"customer": {
"name": "John Doe",
"country": "US"
},
"items": [
{
"name": "Laptop",
"amount": 1200,
"group": "electronics"
},
{
"name": "Mouse",
"amount": 25,
"group": "accessories"
}
]
}
To loop over and evaluate each item in items
, set the Input Field to items
. The node will then process each element in the items
array separately, applying the rules defined in that node.
Example 2: Using Expressions for Preprocessing
You can use GoRules expressions within Input Field to filter or manipulate the data before processing. For example, if you only want to evaluate items that belong to the "accessories" group, you can set the Input Field to:
filter(items, #.group == 'accessories')
This would preprocess the input to include only items where group
is "accessories", so the node will evaluate:
[
{
"name": "Mouse",
"amount": 25,
"group": "accessories"
}
]
Output path
The Output path allows you to define where the output of the evaluation should be stored in the final response. This can be useful for organizing results without overwriting existing fields.
Example:
If the Output path is set to mappedItems
, the node's evaluation output will be saved under mappedItems
in the response. After processing, the result might look like:
{
"cart": {
"customer": {
"name": "John Doe",
"country": "US"
},
"items": [
{
"name": "Laptop",
"amount": 1200,
"group": "electronics"
},
{
"name": "Mouse",
"amount": 25,
"group": "accessories"
}
],
"mappedItems": [
{ "name": "Mouse", "amount": 25, "discount": 5 }
]
}
}
The Loop Mode is indicated by a sync icon located at the top right, just above the node.
Loops availability
Loops are available from:
BRMS | AGENT | Rust | Node.js | Python | Go |
---|---|---|---|---|---|
1.35.0 | 1.8.0 | 0.33.0 | 0.32.0 | 0.31.0 | v0.11.0 |
Summary
Loop Mode provides a powerful way to process arrays, enabling evaluation of each element independently and outputting results as an array. By utilizing the Input Field, you can specify which part of the input should be looped over, and even preprocess data using GoRules expressions to filter or transform it before evaluation. The Output Path allows you to control where the results are stored, ensuring clear and organized output.
These features give you more control over how data is processed and can be applied to a variety of use cases, from shopping carts to bulk data processing.
Updated 4 months ago